This article is currently in the process of being translated into Vietnamese (~49% done).
Code Comments
Khi viết code, rất nhiều ký tự hay từ mà bạn phải nhập sẽ có một ý nghĩa đặt biệt. Ví dụ, bạn sẽ thấy rất nhiều những dòng từ khoá trong C#, hay class, namespace, public và nhiều dòng khác. Những dòng lệnh này cũng như cac biến và phương thức sử dụng trong code bạn cũng sẽ thấy khi biên dịch. C# là một ngôn ngữ cũng khá khắc khe trong việc biên dịch. Tuy nhiên, bạn có thể viết những dòng code bạn thích theo kiểu code comment.
Nếu bạn có kinh nghiệm trong việt viết ghi chú những dòng code trong C# hay bất kỳ ngôn ngữ nào khác, thì những qui tắc viết ghi chú khá nhiều. Chúng ta hãy xem những loại ghi chú nào bạn có thể dùng cho những dòng code C# của bạn.
Ghi chú trên 1 dòng
Loại ghi chú cơ bản nhất trong C# chính là ghi chú trên 1 dòng. Giống như cái tên được đề cập, mọi thông tin đều được đặt trên 1 dòng.
// My comments about the class name could go here...
class Program
{
......
So that's it: Prefix your lines with two forward slashes (//) and your text goes from something the compiler will check and complain about, to something the compiler completely ignores. And while this only applies to the prefixed line, you are free to do the same on the next line, essentially using single-line comments to make multiple comment lines:
// My comments about the class name could go here...
// Add as many lines as you would like
// ...Seriously!
class Program
{
......
Ghi chú trên nhiều dòng
In case you want to write multiple lines of comments, it might make more sense to use the multi-line comment variant that C# offers. Instead of having to prefix every line, you just enter a start and stop character sequence - everything in between is treated as comments:
/*
My comments about the class name could go here...
Add as many lines of comments as you want
...and use indentation, if you want to!
*/
class Program
{
......
Sử dụng cú pháp bắt đầu start sequence theo mẫu ký tự xuyệt sao (/*), viết bất kỳ thông tin nào mà bạn thích theo nhiều dòng, kết thúc start sequence bằng mẫu ký tự (*/). Tức là, thông tin bạn ghi chú sẽ đặt trong (/* ... ghi chú ...*/)
Cũng giống như bất kỳ ngôn ngữ lập trình nào khác, dù bạn ghi chú trên một dòng hay nhiều dòng vẫn còn là 1 vấn đề tranh luận. Theo cá nhân tôi sử dụng cả 2 loại trong các tình huống khác nhau. Tóm lại, tất cả tuỳ thuộc vào bạn!
Ghi chú tài liệu
Những tài liệu cần ghi chú (đang đề cập đến ghi chú trong XML) cũng giống như ghi chú thông thường, nhưng với định dạng XML. Gồm có 2 mẫu ghi chú: 1 dòng và nhiều dòng. Bạn có thể viết giống như thông thường nhưng được thêm những ký tự bổ sung. Ghi chú 1 dòng XML dùng ba dấu "///" thay vì 2, ghi chú đa dòng có thêm 1 dấu * vào phía sao phần bắt đầu ghi chú. Nó giống như:
class User
{
/// <summary>
/// The Name of the User.
/// </summary>
public string Name { get; set; }
/**
* <summary>The Age of the User.</summary>
*/
public string Age { get; set; }
}
Cho dùng bạn sử dụng ghi chú 1 dòng hay nhiều dòng thì nó đều được dùng rất phổ biến.
Documenting your types and their members with documentation comments is a pretty big subject, and therefore it will be covered more in depth in a later article, but now you know how they look!
Code comments & the Task list
If you're using Visual Studio, you can actually get some help tracking your code comments. In the Task List window (access it from the menu View > Task List) your comments will appear if they use the special, but very simple Task List comment syntax:
//TODO: Change "world" to "universe"
Console.WriteLine("Hello, world!");
//HACK: Don't try this at home....
int answerToLife = 42;
So if the single-line comment is immediately followed by TODO or HACK, it will appear in the Task List of Visual Studio, like this:
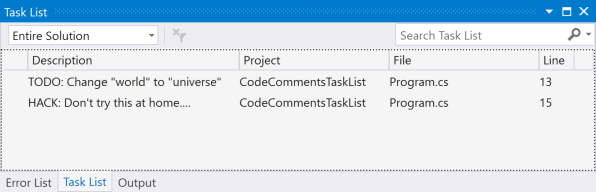
And there are more types - depending on the version of Visual Studio you're using, it will respond to some or all of the following comment tokens:
- TODO
- HACK
- NOTE
- UNDONE
You can even add your own tokens, if you want to - just follow the steps described in this article.
Summary
Code comments are extremely useful in documenting your code or for the purpose of leaving clues to your self or your potential colleagues on how stuff works. As an added benefit, they're great when you need to test something quickly - just copy a line and comment out the original line and you can see how it works now. If you're not happy with the result, you can just delete the new line and uncomment the original line and you're back to where you started.
And don't worry about the end-user snooping through your comments - they are, as already mentioned, completely ignored by the compiler and therefore not in anyway included in your final DLL or EXE file. Code comments are your personal free-space when programming, so use it in any way you want to.